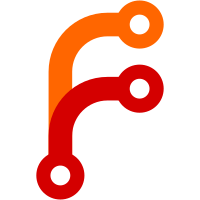
- changed PumpInterface for TimeDateOrTZChangeReceiver changes, and changes on all the pumps (method name and parameters) - some RL changes - added something to profile
44 lines
1,004 B
Java
44 lines
1,004 B
Java
package info.nightscout.androidaps.utils;
|
|
|
|
import java.math.BigDecimal;
|
|
|
|
/**
|
|
* Created by mike on 20.06.2016.
|
|
*/
|
|
public class Round {
|
|
public static Double roundTo(double x, Double step) {
|
|
if (x == 0d) {
|
|
return 0d;
|
|
}
|
|
|
|
//Double oldCalc = Math.round(x / step) * step;
|
|
Double newCalc = BigDecimal.valueOf(Math.round(x / step)).multiply(BigDecimal.valueOf(step)).doubleValue();
|
|
|
|
// just for the tests, forcing failures
|
|
//newCalc = oldCalc;
|
|
|
|
return newCalc;
|
|
}
|
|
|
|
public static Double floorTo(Double x, Double step) {
|
|
if (x != 0d) {
|
|
return Math.floor(x / step) * step;
|
|
}
|
|
return 0d;
|
|
}
|
|
|
|
public static Double ceilTo(Double x, Double step) {
|
|
if (x != 0d) {
|
|
return Math.ceil(x / step) * step;
|
|
}
|
|
return 0d;
|
|
}
|
|
|
|
public static boolean isSame(Double d1, Double d2) {
|
|
double diff = d1 - d2;
|
|
|
|
return (Math.abs(diff) <= 0.000001);
|
|
}
|
|
|
|
}
|